Author : Rahul
Last Modified : 23-Jul-2021
Complexity : Beginner
Implement Telerik Grid with custom Drop Down Filter
Introduction
By default, Telerik Grid shows Textbox for filtering the grid data. Users can write some text into this textbox for filtering the grid data. This implementation shows Drop Down Control instead of Textbox for filtering the grid data. Users can select any value from Drop Down List to filter the grid data.
Implementation
Before starting the implementation of the Telerik Grid, first, we need to download Telerik.Web.UI DLL from Telerik website and add the reference of Telerik.Web.UI DLL into the project. Now put the below code accordingly.
Employee.cs
namespace Grid_POC
{
/// <summary>
/// Class for Employee object
/// </summary>
public class Employee
{
public int EmpId { get; set; }
public string EmpName { get; set; }
}
}
CustomFilterColumn.cs
using System;
using System.Web.UI;
using System.Web.UI.WebControls;
using Telerik.Web.UI;
namespace Grid_POC
{
/// <summary>
/// Class for custom Drop Down Filter
/// </summary>
public class CustomFilterColumn : GridBoundColumn
{
private object listDataSource = null;
/// <summary>
/// Method call on creating Drop Down Filter control within table cell
/// </summary>
/// <param name="cell">Table Cell</param>
protected override void SetupFilterControls(TableCell cell)
{
base.SetupFilterControls(cell);
cell.Controls.RemoveAt(0);
DropDownList dropDownList = new DropDownList();
dropDownList.ID = "dropDownList" + this.DataField;
dropDownList.AutoPostBack = true;
dropDownList.SelectedIndexChanged += new EventHandler(dropDownList_SelectedIndexChanged);
cell.Controls.AddAt(0, dropDownList);
cell.Controls.RemoveAt(1);
dropDownList.DataTextField = this.DataField;
dropDownList.DataValueField = this.DataField;
dropDownList.DataSource = this.ListDataSource;
}
/// <summary>
/// Event called when we select any value from Drop Down List
/// </summary>
/// <param name="sender">sender</param>
/// <param name="e">e</param>
protected void dropDownList_SelectedIndexChanged(object sender, EventArgs e)
{
GridFilteringItem filterItem = (sender as DropDownList).NamingContainer as GridFilteringItem;
if ((sender as DropDownList).SelectedValue == "[All]")
{
filterItem.FireCommandEvent("ClearFilter", new Pair("NoFilter", this.UniqueName));
}
else
{
if (this.DataType == System.Type.GetType("System.Int32") || this.DataType == System.Type.GetType("System.Int16") || this.DataType == System.Type.GetType("System.Int64"))
{
filterItem.FireCommandEvent("Filter", new Pair("EqualTo", this.UniqueName));
}
else
{
filterItem.FireCommandEvent("Filter", new Pair("Contains", this.UniqueName));
}
}
}
/// <summary>
/// Property that contains data source for Drop Down List
/// </summary>
public object ListDataSource
{
get
{
return this.listDataSource;
}
set
{
listDataSource = value;
}
}
/// <summary>
/// Method is used to set current Filter value into Filter Drop Down control
/// </summary>
/// <param name="cell">Table cell</param>
protected override void SetCurrentFilterValueToControl(TableCell cell)
{
base.SetCurrentFilterValueToControl(cell);
DropDownList list = (DropDownList)cell.Controls[0];
if (this.CurrentFilterValue != string.Empty)
{
list.SelectedValue = this.CurrentFilterValue;
}
}
/// <summary>
/// Method is used to get current Filter value from Filter Drop Down control
/// </summary>
/// <param name="cell">Table cell</param>
/// <returns>Current Filter value from Filter Drop Down control</returns>
protected override string GetCurrentFilterValueFromControl(TableCell cell)
{
DropDownList list = (DropDownList)cell.Controls[0];
return list.SelectedValue;
}
/// <summary>
/// Method is used to get Filter Data Field on which filtering is doing
/// </summary>
/// <returns>Filter Data Field</returns>
protected override string GetFilterDataField()
{
return this.DataField;
}
}
}
Grid_CustomFilter.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Grid_CustomFilter.aspx.cs" Inherits="Grid_POC.Grid_CustomFilter" %>
<%@ Register Namespace="Grid_POC" Assembly="Grid_POC" TagPrefix="custom" %>
<%@ Register Namespace="Telerik.Web.UI" Assembly="Telerik.Web.UI" TagPrefix="telerik" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Telerik Grid with custom Drop Down Filter</title>
</head>
<body>
<form runat="server">
<asp:ScriptManager runat="server"></asp:ScriptManager>
<h3>Telerik Grid with custom Drop Down Filter</h3>
<telerik:RadGrid ID="RadGrid1" runat="server" AllowFilteringByColumn="true"
AllowPaging="true" PageSize="5" OnItemCommand="RadGrid1_ItemCommand"
AllowSorting="true" OnNeedDataSource="RadGrid1_NeedDataSource">
<MasterTableView AutoGenerateColumns="False">
<CommandItemTemplate>
<asp:LinkButton runat="server" ID="LinkButton1" Text="Clear Filters"
CommandName="ClearFilters" />
</CommandItemTemplate>
<Columns>
<custom:CustomFilterColumn HeaderText="Employee Id"
DataField="EmpId" UniqueName="EmpId" />
<custom:CustomFilterColumn HeaderText="Employee Name"
DataField="EmpName" UniqueName="EmpName" />
</Columns>
</MasterTableView>
</telerik:RadGrid>
</form>
</body>
</html>
Grid_CustomFilter.aspx.cs
using System;
using System.Collections.Generic;
using System.Data;
using Telerik.Web.UI;
namespace Grid_POC
{
public partial class Grid_CustomFilter : System.Web.UI.Page
{
/// <summary>
/// Page Load Event
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
protected void Page_Load(object sender, EventArgs e)
{
RadGrid1.MasterTableView.DataKeyNames = new[] { "EmpId" };
foreach (GridBoundColumn column in RadGrid1.MasterTableView.Columns)
{
if (column is CustomFilterColumn)
{
(column as CustomFilterColumn).ListDataSource = GetFilterTable(column.DataField);
}
}
}
/// <summary>
/// Event which contains RadGrid Item Commands
/// </summary>
/// <param name="source"></param>
/// <param name="e"></param>
protected void RadGrid1_ItemCommand(object source, GridCommandEventArgs e)
{
switch (e.CommandName)
{
case "ClearFilters":
foreach (GridColumn column in RadGrid1.MasterTableView.Columns)
{
column.CurrentFilterFunction = GridKnownFunction.NoFilter;
column.CurrentFilterValue = String.Empty;
}
RadGrid1.MasterTableView.FilterExpression = String.Empty;
RadGrid1.MasterTableView.Rebind();
break;
}
}
/// <summary>
/// Event called when Data Source is needed
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
protected void RadGrid1_NeedDataSource(object sender, GridNeedDataSourceEventArgs e)
{
RadGrid1.DataSource = GetEmployeeList();
}
/// <summary>
/// Method is used to get Data Table which will bind into Filter Drop Down
/// </summary>
/// <param name="field">Header Field/Property Name</param>
/// <returns>Filter Data Table</returns>
protected DataTable GetFilterTable(string field)
{
DataTable returnTable = new DataTable();
List<object> objList = new List<object>();
Type columnType = Type.GetType("System.String");
List<Employee> empList = GetEmployeeList();
foreach (var item in empList)
{
if (field == "EmpId")
objList.Add(item.EmpId);
else
{
if (!objList.Contains(item.EmpName))
objList.Add(item.EmpName);
}
}
if (objList.Contains(string.Empty))
objList.Remove(string.Empty);
objList.Sort();
objList.Insert(0, "[All]");
returnTable.Columns.Add(field, columnType);
foreach (object obj in objList)
{
returnTable.Rows.Add(obj);
}
return returnTable;
}
/// <summary>
/// Method that return Employee List
/// In Real case this will replace with Database call/Service call
/// </summary>
/// <returns>List of Employees</returns>
private List<Employee> GetEmployeeList()
{
List<Employee> lstEmployee = new List<Employee>();
lstEmployee.Add(new Employee() { EmpId = 1, EmpName = "RahulGoel" });
lstEmployee.Add(new Employee() { EmpId = 2, EmpName = "RajeshSingh" });
lstEmployee.Add(new Employee() { EmpId = 3, EmpName = "RahulGoel" });
lstEmployee.Add(new Employee() { EmpId = 4, EmpName = "Rajesh" });
lstEmployee.Add(new Employee() { EmpId = 5, EmpName = "RajeshSingh" });
lstEmployee.Add(new Employee() { EmpId = 6, EmpName = "Rahul" });
lstEmployee.Add(new Employee() { EmpId = 7, EmpName = "Rahul" });
lstEmployee.Add(new Employee() { EmpId = 8, EmpName = "RahulGoel" });
return lstEmployee;
}
}
}
Output
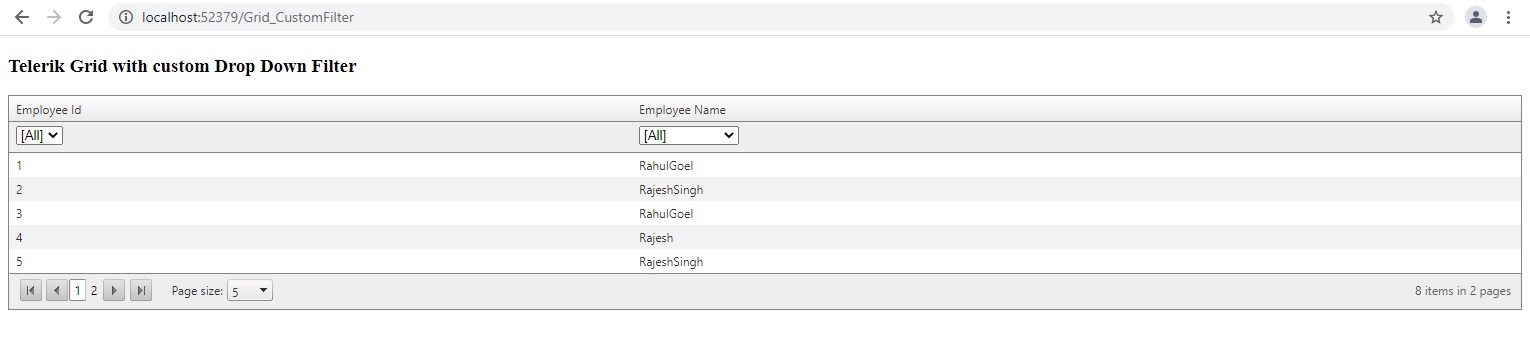